自动化测试jest
##前段测试框架jest
mathers 匹配器
相等
toBe(value): 比较数字、字符串
toEqual(value): 比较对象、数组
toBeNull() 仅当expect返回对象为 null时
toBeUndefined() 仅当返回为 undefined
toBeDefined 和上面的刚好相反,对象如果有定义时1
2
3
4
5
6
7
8
9
10
11
12
13
14
15it("should 3 plus 3 is 6", => {
expect(3+3).toBe(6);
})
test('two plus two', () => {
const value = 2 + 2;
expect(value).toBeGreaterThan(3);
expect(value).toBeGreaterThanOrEqual(3.5);
expect(value).toBeLessThan(5);
expect(value).toBeLessThanOrEqual(4.5);
// toBe and toEqual are equivalent for numbers
expect(value).toBe(4);
expect(value).toEqual(4);
});需要注意的是对于float类型的浮点数计算的时候,需要使用toBeCloseTo而不是 toEqual ,因为避免细微的四舍五入引起额外的问题
1
2
3
4
5test('adding floating point numbers', () => {
const value = 0.1 + 0.2;
//expect(value).toBe(0.3); This won't work because of rounding error
expect(value).toBeCloseTo(0.3); // This works.
});1
2
3
4
5
6
7
8test('null', () => {
const n = null;
expect(n).toBeNull();
expect(n).toBeDefined();
expect(n).not.toBeUndefined();
expect(n).not.toBeTruthy();
expect(n).toBeFalsy();
});包含
toHaveProperty(keyPath, value): 是否有对应的属性
toContain(item): 是否包含对应的值,括号里写上数组、字符串
toMatch(regexpOrString): 括号里写上正则1
2
3
4
5
6
7
8
9
10
11const shoppingList = [
'diapers',
'kleenex',
'trash bags',
'paper towels',
'beer',
];
test('the shopping list has beer on it', () => {
expect(shoppingList).toContain('beer');
});逻辑
toBeTruthy()
toBeFalsy()
在JavaScript中,有六个falsy值:false,0,’’,null, undefined,和NaN。其他一切都是Truthy。toBeGreaterThan(number): 大于
toBeLessThan(number): 小于取反 not
1
2
3
4
5
6
7test('adding positive numbers is not zero', () => {
for (let a = 1; a < 10; a++) {
for (let b = 1; b < 10; b++) {
expect(a + b).not.toBe(0);
}
}
});字符型匹配
使用toMatch匹配规则,支持正则表达式匹配1
2
3
4
5
6
7test('there is no I in team', () => {
expect('team').not.toMatch(/I/);
});
test('but there is a "stop" in Christoph', () => {
expect('Christoph').toMatch(/stop/);
});异常匹配
可以用toThrow测试function是否会抛出特定的异常信息1
2
3
4
5
6
7
8
9
10
11
12function compileAndroidCode() {
throw new ConfigError('you are using the wrong JDK');
}
test('compiling android goes as expected', () => {
expect(compileAndroidCode).toThrow();
expect(compileAndroidCode).toThrow(ConfigError);
// You can also use the exact error message or a regexp
expect(compileAndroidCode).toThrow('you are using the wrong JDK');
expect(compileAndroidCode).toThrow(/JDK/);
});其他
.toBeCalledWith()
.toHaveBeenCalledWith() 使用.toHaveBeenCalledWith以确保模拟函数被调用的具体参数。1
2
3
4
5
6
7test('registration applies correctly to orange La Croix', () => {
const beverage = new LaCroix('orange');
register(beverage);
const f = jest.fn();
applyToAll(f);
expect(f).toHaveBeenCalledWith(beverage);
});.toBeCalledTimes(number)
.toHaveBeenCalledTimes(number)
.toBeCalled()
.toHaveBeenCalled()
.toHaveReturned() 如果你有一个模拟函数,你可以.toHaveReturned用来测试模拟函数成功返回(即,没有抛出错误)至少一次。1
2
3
4
5
6
7test('drinks returns', () => {
const drink = jest.fn(() => true);
drink();
expect(drink).toHaveReturned();
});.toHaveReturnedWith(value) 使用.toHaveReturnedWith以确保模拟函数返回的特定值。
1
2
3
4
5
6
7
8test('drink returns La Croix', () => {
const beverage = {name: 'La Croix'};
const drink = jest.fn(beverage => beverage.name);
drink(beverage);
expect(drink).toHaveReturnedWith('La Croix');
});
测试函数
同步函数
1
2
3
4
5
6
7
8\\s.js
const sum = (a,b) => a + b;
\\_test_/s.test.js
import * as s from '../s'
test("should sum(2,2) is 4",()=>{
except(s.sum(2,2)).toBe(4);
})异步函数
对于异步函数,比如 ajax 请求,测试写法同样容易 待测试文件:client.js1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23\\client.js
export const get = (url, headers = {}) => {
return fetch(url, {
method: 'GET',
headers: {
...getHeaders(),
...headers
}
}).then(parseResponse)
}
\\_test_/client.test.js
import * as client from '../s'
test('fetch by get method', async () => {
expect.assertions(1)
// 测试使用了一个免费的在线 JSON API
const url = 'https://jsonip.com/'
const data = await get(url)
const { about } = data
expect(about).toBe('/about')
})测试的生命周期
- afterAll(fn, timeout): 当前文件中的所有测试执行完成后执行 fn, 如果 fn 是 promise,jest 会等待 timeout 毫秒,默认 5000
- afterEach(fn, timeout): 每个 test 执行完后执行 fn,timeout 含义同上
- beforeAll(fn, timeout): 同 afterAll,不同之处在于在所有测试开始前执行
- beforeEach(fn, timeout): 同 afterEach,不同之处在于在每个测试开始前执行
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23BeforeAll(() => {
console.log('before all tests to excute !')
})
BeforeEach(() => {
c onsole.log('before each test !')
})
AfterAll(() => {
console.log('after all tests to excute !')
})
AfterEach(() => {
console.log('after each test !')
})
Test('test lifecycle 01', () => {
expect(1 + 2).toBe(3)
})
Test('test lifecycle 03', () => {
expect(2 + 2).toBe(4)
})
本文作者 : 对六
原文链接 : http://duiliuliu.github.io/2018/07/01/2018-06-31-自动化测试jest/
版权声明 : 本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明出处!
你我共勉!
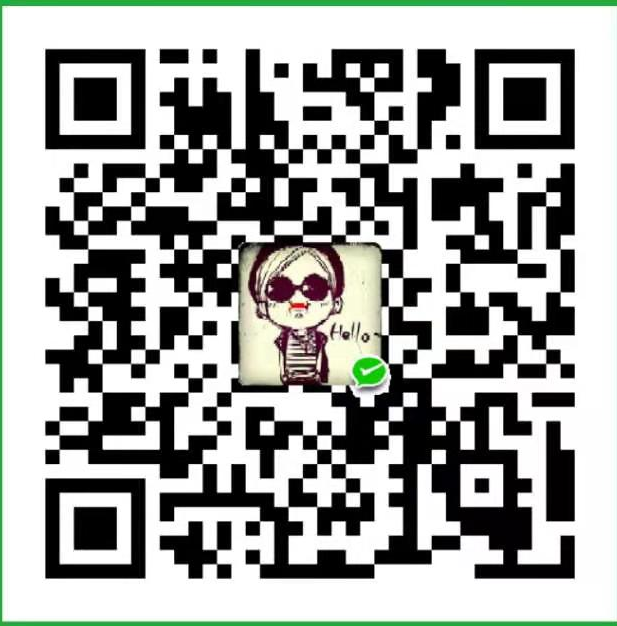
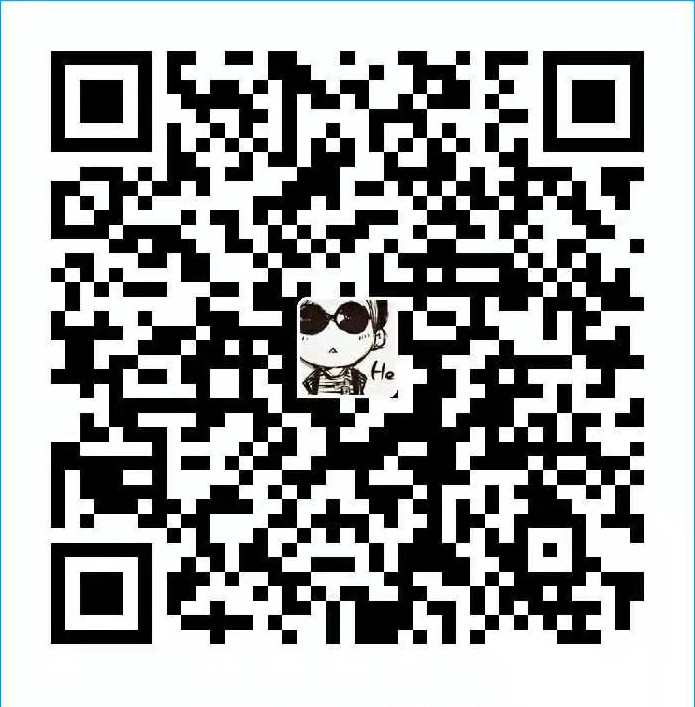