js中forEach与map
js中forEach与map
- 共同点:
- 1、都是循环遍历数组中的每一项。
- 2、forEach()和map()里面每一次执行匿名函数都支持3个参数:数组中的当前项item,当前项的索引index,原始数组input。
- 3、匿名函数中的this都是指Window。
- 区别:
- foreach 无返回值,而map有返回值
- forEach和map还存在一个编程思想的区别,前者是命令式编程,后者是声明式编程,如果项目的风格是声明式的,比如React,那么后者显然更统一。
forEach
语法
1
2
3
4
5
6
7
8
9
10
11
12
13array.forEach(callback(currentValue, index, array){
//do something
}, this)
//或者
array.forEach(callback(currentValue, index, array){
//do something
})
//或者 lambda表达式,较简便
array.forEach(element => {
});callback 函数会被依次传入三个参数:
- 数组当前项的值
- 数组当前项的索引
- 数组对象本身
如果给forEach传递了thisArg参数,当调用时,它将被传给callback 函数,作为它的this值。否则,将会传入 undefined 作为它的this值。
因为js中的数组时引用类型,所以可以用foreach来更新数组
注意
在使用forEach()时候,如果数组在迭代的视乎被修改,则其他元素会被跳过。因为 forEach()不会在迭代之前创建数组的副本。
1
2
3
4
5
6
7
8
9
10var words = ["1","2","3","4"];
words.forEach(word => {
console.log(word);
if (word === "2"){
words.shift();
}
});
// 1
// 2
// 4
map
语法
1
2
3
4
5
6
7
8
9
10var new_array = arr.map(callback[, thisArg])
//或者 有返回值
arr[].map(function(value,index,array){
//do something
return XXX
})参数:value数组中的当前项,index当前项的索引,array原始数组;
如:
1
2
3
4
5
6var ary = [12,23,24,42,1];
var res = ary.map(function (item,index,input) {
return item*10;
})
console.log(res);//-->[120,230,240,420,10]; 原数组拷贝了一份,并进行了修改
console.log(ary);//-->[12,23,24,42,1]; 原数组并未发生变化如:反转字符串:
1
2
3
4var str = '12345';
Array.prototype.map.call(str, function(x) { //同时利用了call()方法
return x;
}).reverse().join('');注意
1
["1", "2", "3"].map(parseInt); //结果 [1, NaN, NaN]
如果想得到[1, 2,3]应该这么做1
2
3
4
5function returnInt(element){
return parseInt(element,10);
}
["1", "2", "3"].map(returnInt);这主要是因为 parseInt()默认有两个参数,第二个参数是进制数。当parsrInt没有传入参数的时候,而map()中的回调函数时候,会给它传三个参数,第二个参数就是索引,明显不正确,所以返回NaN了。
兼容写法
不管是forEach还是map在IE6-8下都不兼容(不兼容的情况下在Array.prototype上没有这两个方法),那么需要我们自己封装一个都兼容的方法,代码如下:
1 | /** |
本文作者 : 对六
原文链接 : http://duiliuliu.github.io/2018/06/01/2018-06-01-js中forEach与map/
版权声明 : 本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明出处!
你我共勉!
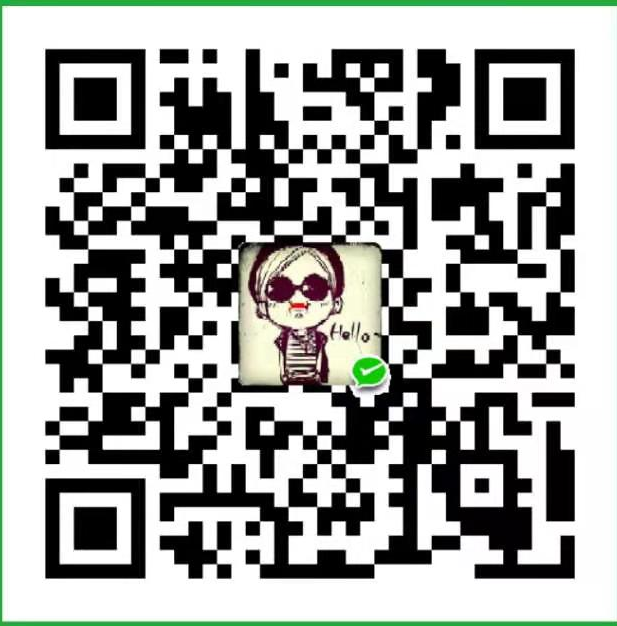
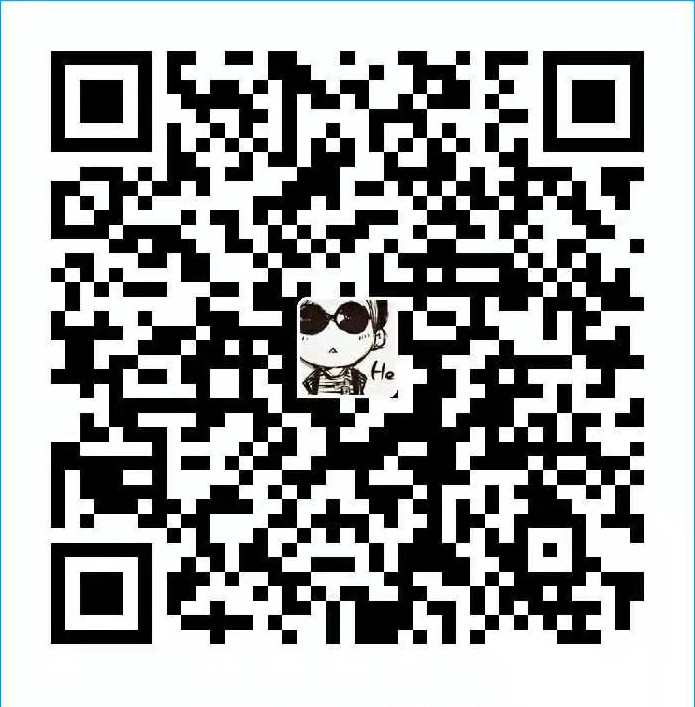